03 Dec 2012 A short list of most useful Git commands to work with git tags. These should cover 90% of your needs.
### TAGS
#create tag (annotated = with extra meta information)
git tag -a ver1.4 -m 'my version 1.4'
#list tags
git tag
#delete tag
git tag -d XXX
#delete remote tag
#You just need to push an 'empty' reference to the remote tag name:
git push origin :tagname
#push tags to remote
#specific tag:
git push origin v1.5
#all tags:
git push origin --tags
01 Nov 2012 Keeps the names of all public classes in the specified package:
-keep public class com.myapp.customcomponents.*
The following configuration keeps the names of all public classes in the specified package and its subpackages:
-keep public class com.myapp.customcomponents.**
The following configuration keeps the names of all public/protected classes/fields/methods in the specified package and its subpackages:
-keep public class com.myapp.customcomponents.** {
public protected *;
}
30 Oct 2012 I hate facebook Engineers. Yes, you heard me right. I hate them. Well, maybe not them but their hairy pointy managers and bosses who... wait a second, isn't facebook "famous" for being all staffed with engineers? So yes, I hate facebook Engineers
Now you will say but why, they are mostly smart guys and facebook is so spectafackamazing. WHY?!?... Well, for one their mobile SDK suck. Full of bugs, crashes and other pleasantries. I did at least 5 updates solely because there was some problem with Facebook and the most interesting part of that is that the updates were required after some change that was made that broke things. The integration is working 100% for 4 month and then one day goes KABOOM.
They are all warm and fuzzy now, trying to get to mobile developers, well you got under my skin for sure guys. But even now, when all news are raving how they redesigned and updated and what not their portals for mobile developers who use their SDK to integrate with them they still have problems. Just couple days ago when they started pushing into every possible media hole the news that they got the new SDK and blahblahblah
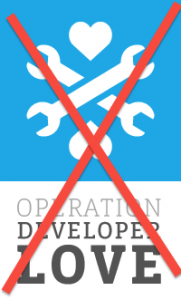
reserved but intrigued (can it be that FINALLY they got the SDK to a decent shape?!?) off I went to check it. For a long time I have been using the latest clone of their github repository and I decided to see whether it is different from the repository - indeed it was. Ok, I thought I should use the SDK and off I went to integrate it within my app just to see that it crashes 100% of the time with SSL error. Damn I though and went back to github's repo which not surprisingly did not have this problem.
That's not funny.... It never was. Seriously - pull your shit together! I don't need and don't want to spend 2–5 hours every few months to work around yet another problem you have introduced.
23 Oct 2012 To remove inactive ports:
sudo port uninstall inactive
To remove downloaded distfiles with:
sudo port clean --all installed
To remove orphaned (not referenced and not anymore used ports) you can use an external tool port_cutleaves
First install the tool using MacPorts
sudo port install port_cutleaves
Then run it
sudo port_cutleaves
14 Sep 2012 The following crash was cripping into the reports. There is no usage of the webview directly, but there is a usage of the webview and thus related stuff in the ads.
java.lang.NullPointerException
at android.webkit.WebViewDatabase.getCacheTotalSize(WebViewDatabase.java:815)
at android.webkit.CacheManager.trimCacheIfNeeded(CacheManager.java:548)
at android.webkit.WebViewWorker.handleMessage(WebViewWorker.java:190)
at android.os.Handler.dispatchMessage(Handler.java:99)
at android.os.Looper.loop(Looper.java:123)
at android.os.HandlerThread.run(HandlerThread.java:60)
The problem is the following code:
long getCacheTotalSize() {
long size = 0;
Cursor cursor = null;
final String query = "SELECT SUM(contentlength) as sum FROM cache";
try {
cursor = mCacheDatabase.rawQuery(query, null);
if (cursor.moveToFirst()) {
size = cursor.getLong(0);
}
} catch (IllegalStateException e) {
Log.e(LOGTAG, "getCacheTotalSize", e);
} finally {
if (cursor != null)
cursor.close();
}
return size;
}
The NullPointerException that happens should in general not happen but sometimes the storage gets corrupted and then the cache database is null when it is called and it crashes.
The fix would be to check for this problem and then only use WebView and/or call the ads if there is no problem with the WebViewDatabase
WebViewDatabase webViewDB = WebViewDatabase.getInstance(this);
if (webViewDB != null) {
// do what you wanted to do with the WebView
}
06 Sep 2012 Most people heard about the 80/20 rule.
For those who didn't it basically says that you do 80% of work in 20% time and then 80% of time is spent on the remaining 20% to finish the project/product/service.
BUT
It is these 20% of polish that will ultimately get you the 80% of the market. Because the teams that spent 80% (or even more) on the last 20% of polish to their product and/or service are the teams that usually produce the product/service that wins the major of the market. And this is all that usually matters – to own the majority of the market, to be the leader or in the leader pack in the market.
This is not a recipe for success of course. There are polished and good products that fail. Speaking in math terms, this is rather the necessary, than sufficient condition for a successful product and/or service.
06 Sep 2012 Garbage collection in Eclipse
There are several GC options available for eclipse's optimization. Try which one works best for you
The (original) copying collector (Enabled by default). When this collector kicks in, all application threads are stopped, and the copying collection proceeds using one thread (which means only one CPU even if on a multi-CPU machine). This is known as a stop-the-world collection, because basically the JVM pauses everything else until the collection is completed.
The parallel copying collector (Enabled using -XX:+UseParNewGC). Like the original copying collector, this is a stop-the-world collector. However this collector parallelizes the copying collection over multiple threads, which is more efficient than the original single-thread copying collector for multi-CPU machines (though not for single-CPU machines). This algorithm potentially speeds up young generation collection by a factor equal to the number of CPUs available, when compared to the original singly-threaded copying collector.
The parallel scavenge collector (Enabled using -XX:UseParallelGC). This is like the previous parallel copying collector, but the algorithm is tuned for gigabyte heaps (over 10GB) on multi-CPU machines. This collection algorithm is designed to maximize throughput while minimizing pauses. It has an optional adaptive tuning policy which will automatically resize heap spaces. If you use this collector, you can only use the the original mark-sweep collector in the old generation (i.e. the newer old generation concurrent collector cannot work with this young generation collector).
Speeding up Eclipse
If you want more information on how to speed up eclipse on OS X – read this post: [link id='240' text='How To speed up Eclipse on OS X']